Log4j is one very popular logging framework which is widely used by a lot java applications such as hadoop, tomcat. here is quick tutorial for programmers to setup the log4j and write some very basic logging applications.
first, create a java application and download reference the log4j jar dependency, if you use maven, just add log4j to the dependency list in your pom.xml.
I have the maven eclipse plugin, so I can create one simple maven project.
give it a groupid and artifact id
right click pom.xml, and chose the maven menu, then add dependency, search log4j. and click to add one latest version to the dependency.
then the pom file loos like this
now the project has the log4j jar in the maven’s dependencies folder.
Now let’s create one simple class with one main method to do the logging.
basically, we just create one logger by calling the logmanaager,getlogger. then can log out some message by using different level settings.
now we can tell from the error message , there is no logger for com.androidyou. so we need to put some instruction into the log4j.properties , basically tell the runtime which logger I should use and for a giving logger, what’s the logging level. for each given logger, what appender we should use, then the properties for the appenders.
put the log4j.debug=true, you will see more verbal messages. it tells us that no resource file found (by default it’s the log4j.properties.) we can override the resource file to whaterve file we have by passing the locatio to the log4j.configuration property.
Now we just create a very basic log4j.properties file under any classpath folder, I just put it under src/main/resources folder.
# console is set to be a ConsoleAppender. log4j.appender.console.layout=org.apache.log4j.PatternLayout |
run again, you can see the loading process, our resource file log4j.properties get picked up, and our logger also identified and level debug is assigned
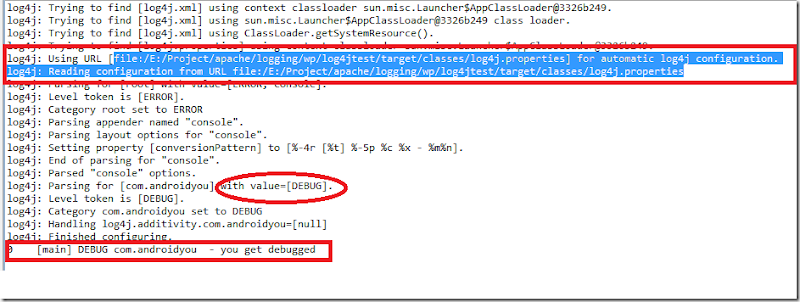
for the logging configuration, there are different levels. here is the list, I checked the code declaration
Also there are a lot built-in appenders.
here is one example that we change the consoleappender to dailyrollingfileappender, which rotate the file by time setting.
log4j.logger.com.androidyou=DEBUG # console is set to be a DailyRollingFileAppender. log4j.appender.df.DatePattern='.'yyyy-MM-dd-HH log4j.appender.df.layout=org.apache.log4j.PatternLayout |
Besides the log4j. slf4j is another popular logging framwrok, it’s a façade layer which works as a separate stable interface, slf4j-log4j is a implementation. so code is almos the same, you only need change the pacakge namer from log4j to slf4j.
for the pom, API is the core library, log4j is the implementation. we can simply copy another impementation like no-op to the classpath, then no log will be gerated.
Also we can send the messages to the ActiveMQ Topics.
Change the log4.properties to add JMSappender.
log4j.rootLogger=ERROR, jms log4j.appender.jms=org.apache.log4j.net.JMSAppender |
then define one jndi.properties file and put it under any classpath folder.
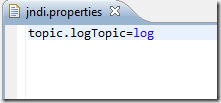
then reference all the jars under activemq lib for safe.
then you can see the messages in the topic we created.
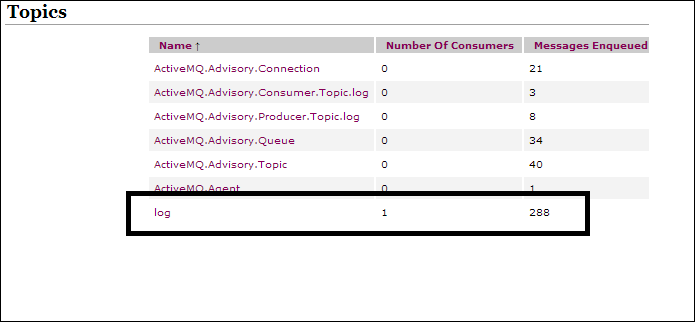
under activemq examples, run ant consumer to consumer the topics,
